Cosmos Staking API
1 Stake
Staking ATOM through the InfStones Safe Stake SDK involves a straightforward process: users stake ATOM and are provided with an activity ID. This activity ID can be utilized to access the raw transaction which users can then sign and broadcast to complete the staking process seamlessly.
1.1 Stake
Through stake
API, users can stake a specified amount of ATOM and get the activity ID.
Request
curl -X 'POST' \
'https://stakingapi.infstones.com/cosmos/stake' \
-H 'x-api-key: <access_token>' \
-H 'Content-Type: application/json' \
-d '{
"wallet": "<your_cosmos_wallet_address>",
"amount": "<stake_amount>"
}'
<access_token>
should be asked from InfStones. For more details, please check https://docs.infstones.com/docs/get-started#1-create-access-token.
Response
{
"data": "2df44310-4462-434d-add4-93f6ae175548"
}
1.2 Get Raw Transaction
Open GraphQL Playground, users can retrieve the raw transaction associated with the activity ID from 1.1 Stake
.
Step 1: Input your access token in the Headers
section.
Step 2: Input the activity ID and run the command, you will get the response with raw_tx
.
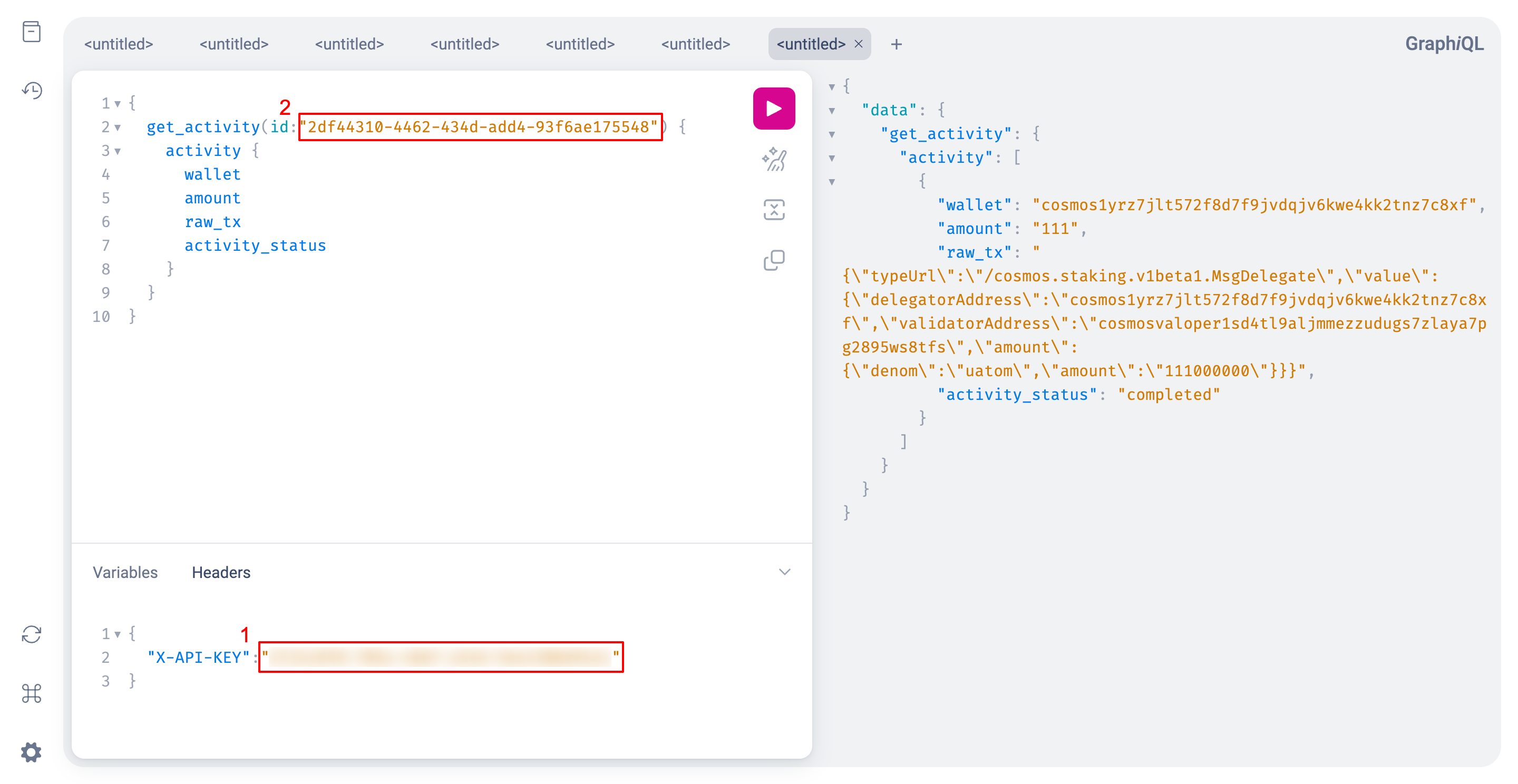
Request
{
get_activity(id:"2df44310-4462-434d-add4-93f6ae175548") {
activity {
wallet
amount
raw_tx
activity_status
}
}
}
Response
{
"data": {
"get_activity": {
"activity": [
{
"wallet": "cosmos1yrz7jlt572f8d7f9jvdqjv6kwe4kk2tnz7c8xf",
"amount": "111",
"raw_tx": "{\"typeUrl\":\"/cosmos.staking.v1beta1.MsgDelegate\",\"value\":{\"delegatorAddress\":\"cosmos1yrz7jlt572f8d7f9jvdqjv6kwe4kk2tnz7c8xf\",\"validatorAddress\":\"cosmosvaloper1sd4tl9aljmmezzudugs7zlaya7pg2895ws8tfs\",\"amount\":{\"denom\":\"uatom\",\"amount\":\"111000000\"}}}",
"activity_status": "completed"
}
]
}
}
}
The activity status
completed
means the transaction details are fully prepared and ready for the next stepSign Transaction and Broadcast
.
1.3 Sign Transaction and Broadcast
Users can sign the raw transaction using their own wallet's private key and broadcast it to complete the staking process.
Follow the instructions to get your Cosmos Fast API Tendermint endpoint.
import { coins, makeCosmoshubPath } from "@cosmjs/amino"
import { DirectSecp256k1HdWallet, Registry } from "@cosmjs/proto-signing"
import {
SigningStargateClient,
calculateFee,
GasPrice,
StargateClient,
} from "@cosmjs/stargate"
const mnemonic = "<your_cosmos_wallet_mnemonic>" // Replace this with your Cosmos wallet mnemonic
const wallet = await DirectSecp256k1HdWallet.fromMnemonic(mnemonic)
const gasPrice = GasPrice.fromString("0.0025uatom")
const [account] = await wallet.getAccounts()
const rpcEndpoint = "<infstones_rpc_api_endpoint>" // Replace this with your Cosmos Fast API Tendermint endpoint
const client = await SigningStargateClient.connectWithSigner(
rpcEndpoint,
wallet,
{ gasPrice: gasPrice }
)
// stake raw tx
const rawTx = {
typeUrl: "/cosmos.staking.v1beta1.MsgDelegate",
value: {
delegatorAddress: "<delegator_address>",
validatorAddress: "<validator_address>",
amount: {
denom: "uatom",
amount: "<amount>", // Replace this with the amount in the response of step 1.2
},
},
}
const memo = "stake"
const gasEstimation = await client.simulate(account.address, [rawTx], memo)
const fee = calculateFee(Math.round(gasEstimation * 1.3), gasPrice)
const result = await client.signAndBroadcast(
account.address,
[rawTx],
fee,
memo
)
console.log("Successfully broadcasted:", result)
2 Unstake
2.1 Unstake
Unstaking ATOM through the InfStones Safe Stake SDK involves a straightforward process: users unstake ATOM and are provided with an activity ID. This activity ID can be utilized to access the raw transaction which users can then sign and broadcast to complete the unstaking process seamlessly.
Request
curl -X 'POST' \
'https://stakingapi.infstones.com/cosmos/unstake' \
-H 'x-api-key: <access_token>' \
-H 'Content-Type: application/json' \
-d '{
"wallet": "<your_cosmos_wallet_address>",
"amount": "<unstake_amount>"
}'
Response
{
"data": "af41e0bb-960b-4efa-8727-39a20d6b6b09"
}
2.2 Get Raw Transaction
Open GraphQL Playground, users can retrieve the raw transaction associated with the activity ID from 2.1 Unstake
.
Request
{
get_activity(id:"88765143-bdef-489a-8d6b-9e8d5ce3438f") {
activity {
wallet
amount
raw_tx
activity_status
}
}
}
Response
{
"data": {
"get_activity": {
"activity": [
{
"wallet": "cosmos1yrz7jlt572f8d7f9jvdqjv6kwe4kk2tnz7c8xf",
"amount": "0.1",
"raw_tx": "{\"typeUrl\":\"/cosmos.staking.v1beta1.MsgUndelegate\",\"value\":{\"delegatorAddress\":\"cosmos1wlf0uk0yfr8rz3un4r7dq5wsydvnqgg5d8ll75\",\"validatorAddress\":\"cosmosvaloper1sd4tl9aljmmezzudugs7zlaya7pg2895ws8tfs\",\"amount\":{\"denom\":\"uatom\",\"amount\":\"100000\"}}}",
"activity_status": "completed"
}
]
}
}
}
The activity status
completed
means the transaction details are fully prepared and ready for the next stepSign Transaction and Broadcast
.
2.3 Sign Transaction and Broadcast
Users can sign the raw transaction using their own wallet's private key and broadcast it to complete the unstake process.
import { coins, makeCosmoshubPath } from "@cosmjs/amino"
import { DirectSecp256k1HdWallet, Registry } from "@cosmjs/proto-signing"
import {
SigningStargateClient,
calculateFee,
GasPrice,
StargateClient,
} from "@cosmjs/stargate"
const mnemonic = "<your_cosmos_wallet_mnemonic>"// Replace this with your cosmos wallet mnemonic
const wallet = await DirectSecp256k1HdWallet.fromMnemonic(mnemonic)
const gasPrice = GasPrice.fromString("0.0025uatom")
const [account] = await wallet.getAccounts()
const rpcEndpoint = "<infstones_rpc_api_endpoint>"// Replace this with your cosmos Fast API Tendermint endpoint
const client = await SigningStargateClient.connectWithSigner(
rpcEndpoint,
wallet,
{ gasPrice: gasPrice }
)
// unstake raw tx
const rawTx = {
typeUrl: "/cosmos.staking.v1beta1.MsgUndelegate",
value: {
delegatorAddress: "<delegator_address>",
validatorAddress: "<validator_address>",
amount: {
denom: "uatom",
amount: "<amount>", // Replace this with the amount in the response of step 2.2
},
},
}
const memo = "unstake"
const gasEstimation = await client.simulate(account.address, [rawTx], memo)
const fee = calculateFee(Math.round(gasEstimation * 1.3), gasPrice)
const result = await client.signAndBroadcast(
account.address,
[rawTx],
fee,
memo
)
console.log("Successfully broadcasted:", result)
3 Claim Reward
Claiming reward through the InfStones Safe Stake SDK involves a straightforward process: users claim reward and are provided with an activity ID. This activity ID can be utilized to access the raw transaction which users can then sign and broadcast to complete the claim reward process seamlessly.
3.1 Claim Reward
Through claim
API, users can claim reward using their <access_token>
and get the activity ID.
Request
curl -X 'POST' \
'https://stakingapi.infstones.com/cosmos/claim' \
-H 'x-api-key: <access_token>' \
-H 'Content-Type: application/json' \
-d '{
"wallet": "<your_cosmos_wallet_address>"
}'
Response
{
"data": "e8cda042-5b56-4af9-bebf-a99a8717d219"
}
3.2 Get Raw Transaction
Open GraphQL Playground, users can retrieve the raw transaction associated with the activity ID from 3.1 Claim Reward
.
Request
{
get_activity(id:"e8cda042-5b56-4af9-bebf-a99a8717d219") {
activity {
wallet
amount
raw_tx
activity_status
}
}
}
Response
{
"data": {
"get_activity": {
"activity": [
{
"wallet": "cosmos1yrz7jlt572f8d7f9jvdqjv6kwe4kk2tnz7c8xf",
"amount": "0.002960",
"raw_tx": "{\"typeUrl\":\"/cosmos.distribution.v1beta1.MsgWithdrawDelegatorReward\",\"value\":{\"delegatorAddress\":\"cosmos1wlf0uk0yfr8rz3un4r7dq5wsydvnqgg5d8ll75\",\"validatorAddress\":\"cosmosvaloper1sd4tl9aljmmezzudugs7zlaya7pg2895ws8tfs\"}}",
"activity_status": "completed"
}
]
}
}
}
The activity status
completed
means the transaction details are fully prepared and ready for the next stepSign Transaction and Broadcast
.
3.3 Sign Transaction and Broadcast
Users can sign the raw transaction using their own wallet's private key and broadcast it to complete the claim reward process.
import { coins, makeCosmoshubPath } from "@cosmjs/amino"
import { DirectSecp256k1HdWallet, Registry } from "@cosmjs/proto-signing"
import {
SigningStargateClient,
calculateFee,
GasPrice,
StargateClient,
} from "@cosmjs/stargate"
const mnemonic = "<your_cosmos_wallet_mnemonic>"// Replace this with your cosmos wallet mnemonic
const wallet = await DirectSecp256k1HdWallet.fromMnemonic(mnemonic)
const gasPrice = GasPrice.fromString("0.0025uatom")
const [account] = await wallet.getAccounts()
const rpcEndpoint = "<infstones_rpc_api_endpoint>"// Replace this with your cosmos Fast API Tendermint endpoint
const client = await SigningStargateClient.connectWithSigner(
rpcEndpoint,
wallet,
{ gasPrice: gasPrice }
)
// claim raw tx
const rawTx = {
typeUrl: "/cosmos.distribution.v1beta1.MsgWithdrawDelegatorReward",
value: {
delegatorAddress: "<delegator_address>",
validatorAddress: "<validator_address>",
},
}
const memo = "claim"
const gasEstimation = await client.simulate(account.address, [rawTx], memo)
const fee = calculateFee(Math.round(gasEstimation * 1.3), gasPrice)
const result = await client.signAndBroadcast(
account.address,
[rawTx],
fee,
memo
)
console.log("Successfully broadcasted:", result)
Updated 25 days ago